Laziness in C# - Sieve of Eratosthenes
After watching "Laziness in Python" YouTube video, I decided to check if it is possible to implement the same algorithm in C# using the yield statement.
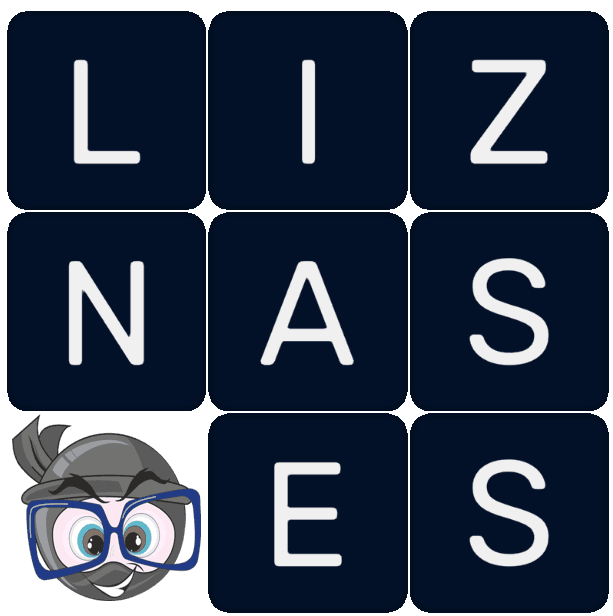
My version uses .NET 7, and I kept the function and variable names the same as in the video.
IEnumerable<int> Nats(int n)
{
yield return n;
foreach (var next in Nats(n + 1))
yield return next;
}
IEnumerable<int> Sieve(IEnumerable<int> s)
{
var n = s.First();
yield return n;
foreach (var i in Sieve(s.Where(x => x % n != 0)))
yield return i;
}
int cnt = 1;
var p = Sieve(Nats(2));
foreach (var i in p.Take(100))
Console.WriteLine($"Prime #{cnt++}: {i}");
It works, but it can calculate only 793 primes on .NET 7, and then it throws a StackOverflow exception.
I changed the Nats function slightly so it didn't use recursion and calculated 7054 primes (the last one being 71257) before I ran out of patience and stopped the program.
IEnumerable<int> Nats(int n)
{
while (true)
yield return n++;
}
You can download and run the code here.
Thank you, Computerphile, for all the great videos!